Trusted and Supported by businesses across the world
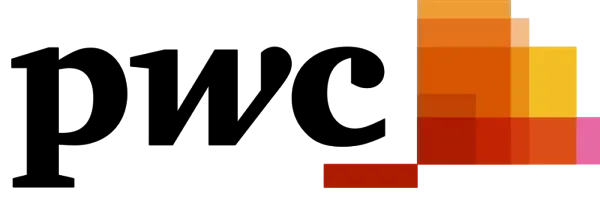

How to Use AI Audio Summary API
Automatically summarize long audio files into concise, easy-to-read text.
Unlock the power of artificial intelligence to transform your audio content into written summaries.
With ScreenApp's AI Audio Summary API, you can:
- Generate human-readable summaries from any audio file.
- Extract key points, insights, and actionable information.
- Save time and effort in reviewing and analyzing audio content.
Our API is designed to be:
- Accurate: Leverages advanced AI algorithms to deliver highly precise summaries.
- Fast: Generates summaries within seconds, making it efficient for large-scale processing.
- Versatile: Supports a wide range of audio formats and languages.
Benefits of AI Audio Summary API
Time-Saving and Efficiency
Automate audio transcription, summarization, and extraction of key insights, saving developers countless hours of manual labor.
Enhanced User Experience
Provide users with concise and informative audio summaries, improving app engagement and satisfaction.
Scalability and Flexibility
Easily integrate the API into existing applications, allowing for seamless audio processing and summarization at scale.
Accurate and Comprehensive
Leverage advanced AI algorithms to generate highly accurate audio summaries that capture the essence of any audio content.
Empowering Developers
Unlock new possibilities for developers by enabling the creation of innovative audio-based applications and services.
.webp)
Online Screen Recorder
Capture your screen and camera in a click without a watermark, including any Teams, Meet, Zoom, or Webex Call.
.webp)
Transcribe in a Flash
Transcribe any video or audio with AI and without lifting a finger with 99% accuracy and lightning speed.
.webp)
Summarize Recordings with AI
Save time and effort. Get an AI-generated summary automatically and focus on what matters.
.webp)
Automatic Notes with AI
Turn your videos and audio into skimmable notes. Click to the part you want to watch.
.webp)
Chat to Your Recordings
Instantly extract action items, decisions, and insights from your recordings. It's like talking to somebody who has watched the videos for you.
.webp)
Instantly Record Audio and Video
Record your audio and Video with 1 click directly from your browser.
.webp)
Translate Videos with AI
Translate Understand any video or audio in over 50 languages.
.webp)
Upload any Video or Audio
Upload any video or audio file for transcription, summaries and notes.
Who is AI Audio Summary API for?
Who is ScreenApp's AI Audio Summary API Perfect for?
Developers, content creators, and businesses looking to:
- Extract key insights and summaries from audio recordings
- Create automatically-generated transcripts and summaries
- Enhance accessibility for audio content through transcripts
- Process large volumes of audio content efficiently
- Integrate AI-powered audio analysis into their workflow
- Provide personalized audio summaries to users
- Gain a deeper understanding of their audio content
.webp)
What is ScreenApp's AI Audio Summary API?
Our API uses the latest AI models to automatically summarize audio content, saving you time and resources.
How does it work?
Simply send us your audio file and our API will return a concise, text-based summary.
What file formats are supported?
We support most common audio formats, including MP3, WAV, and AAC.
What languages are supported?
Our API currently supports English, Spanish, French, and German.
Can I customize the summary length?
Yes, you can specify the desired summary length when making the API call.
Is the API easy to use?
Yes, our API is designed to be developer-friendly with clear documentation and code samples.
What is the pricing?
We offer flexible pricing plans to suit your needs, starting from a free tier for low-volume usage.
Is the API secure?
Yes, our API uses secure protocols to ensure the privacy and confidentiality of your audio data.
Can I try the API before I commit?
Yes, we offer a free trial so you can test the API and see its capabilities firsthand.
How can I get started with the API?
Visit our developer portal for detailed documentation and code samples to get started with our AI Audio Summary API.